React 父组件给 props.children 组件传参
# 父组件给 props.children 组件传参
在 React
组件中向 props.children
传递数据是设计 ButtonGroup
/CheckboxGroup
等组件时常用的技巧, 我们都知道在 React
组件中向子组件传递数据很容易,但是如何向 props.children
传递数据呢?
# 向子组件传递数据
向子组件传递数据很容易,我们只需要将数据放到子组件的 props
里就行了,例如:
点击查看代码
const Checkbox = (props) => {
const { children, ...rest } = props
return (
<label>
<input type="checkbox" {...rest} />
{children}
</label>
)
}
const CheckboxGroup = (props) => {
const { selected = [], group = [] } = props
return (
<Fragment>
{
group.map(
value => (
<Checkbox
checked={selected.indexOf(value) > -1}
key={value}
>
{value}
</Checkbox>
),
)
}
</Fragment>
)
}
render(
<div>
<CheckboxGroup
group={[1, 2, 3]}
selected={[1, 3]}
/>
</div>,
document.getElementById('app'),
)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
在 CheckboxGroup
组件中,我们把 checked
以及 children
传给了子组件Checkbox
。这样的确是可行的,但是这样会导致父组件的配置越来越臃肿,因为我们需要在 CheckboxGroup
上设置一些额外的配置来控制子组件 Checkbox
的样式与行为。为了避免这种情况,我们需要将 CheckboxGroup
和 Checkbox
拆开,Checkbox
的 props
用来控制自己独立的样式、行为等,CheckboxGroup
的 props
主要用来控制子组件是不是全选、哪些选中、自己的样式等,主流的组件库通常这样设计这类组件的API的:
<CheckboxGroup>
<Checkbox>1</Checkbox>
<Checkbox checked>2</Checkbox>
<Checkbox>3</Checkbox>
</CheckboxGroup>
2
3
4
5
这样使用起来更加灵活直观。为了达到这种效果,我们就需要在 CheckboxGroup
内向 props.children
传递数据。下面我们来看看有哪些常用的方法吧!
# React.cloneElement
我们可以借助一个 React
的一个顶层 API
(React.CloneElement
) 来动态的修改 children
的 props
:
点击查看代码
const Checkbox = (props) => {
const { children, ...rest } = props
return (
<label>
<input type="checkbox" {...rest} />
{children}
</label>
)
}
const CheckboxGroup = (props) => {
const { selected = [], children } = props
return (
<Fragment>
{
// children 不是数组我们需要用 React.Children.map 来遍历
// 或者把它转成数组
React.Children.map(children, (child) => {
if (!React.isValidElement(child)) {
return null
}
// 这里我们通常还会判断 child 的类型来确定是不是要传递相应的数据,这里我就不做了
const childProps = {
...child.props,
checked: selected.findIndex(
value => value.toString() === child.props.children,
) > -1,
}
return React.cloneElement(child, childProps)
})
}
</Fragment>
)
}
render(
<div>
<CheckboxGroup
selected={[1, 2]}
>
<Checkbox>1</Checkbox>
<Checkbox>2</Checkbox>
<Checkbox>3</Checkbox>
</CheckboxGroup>
</div>,
document.getElementById('app'),
)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
<CheckboxGroup
selected={[1, 2]}
>
<Fragment>
<Checkbox>1</Checkbox>
<Checkbox>2</Checkbox>
<Checkbox>3</Checkbox>
</Fragment>
</CheckboxGroup>
2
3
4
5
6
7
8
9
因为这时我们修改的就是 Fragment
的 props
了,而不是 Checkbox
的 props
。下面我们来看看另外一种可以摆脱这种困扰的方法。
# renderProps/renderCallback
这里我们的 CheckboxGroup
可以接受一个函数,把子元素需要的数据放在该函数的参数列表里,然后动态的渲染子元素。我们直接来看实现:
点击查看代码
const Checkbox = (props) => {
const { children, ...rest } = props
return (
<label>
<input type="checkbox" {...rest} />
{children}
</label>
)
}
const CheckboxGroup = (props) => {
const { selected = [], children } = props
return (
<Fragment>
{
children(selected)
}
</Fragment>
)
}
render(
<div>
<CheckboxGroup
selected={[1, 2]}
>
{
selected => [1, 2, 3].map(value => (
<Checkbox
key={value}
checked={selected.indexOf(value) > -1}
>
{value}
</Checkbox>
))
}
</CheckboxGroup>
</div>,
document.getElementById('app'),
)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
这种方法可以摆脱 cloneElement
带来的嵌套失效的问题,但是如果使用这种方式不注意拆分的话容易写出下面这种嵌套很深的代码:
<A>
{() => (
<B>
{() => (
<C>
{() => <D />}
</C>
)}
</B>
)}
</A>
2
3
4
5
6
7
8
9
10
11
有没有什么更好的办法呢?有的,我们可以借助 Context
来共享数据。
# Context
当然使用 Context
或者其它状态管理工具逻辑都是一样的,我就不多说了。
点击查看代码
const checkboxContext = createContext([])
const Checkbox = (props) => {
const { children, ...rest } = props
const selected = React.useContext(checkboxContext)
return (
<label>
<input
type="checkbox"
checked={selected.findIndex(
value => value.toString() === children,
) > -1}
{...rest}
/>
{children}
</label>
)
}
const CheckboxGroup = (props) => {
const { selected = [], children } = props
const { Provider } = checkboxContext
return (
<Provider value={selected}>
{children}
</Provider>
)
}
render(
<div>
<CheckboxGroup
selected={[1, 3]}
>
<Checkbox>1</Checkbox>
<Checkbox>2</Checkbox>
<Checkbox>3</Checkbox>
</CheckboxGroup>
</div>,
document.getElementById('app'),
)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
这里我们就不会有嵌套带来的各种各样的问题。
# 示例
点击查看代码
class Mouse extends React.Component {
state = {
clientX: 0,
clientY: 0
}
componentDidMount() {
document.addEventListener('mousemove', ({ clientX, clientY }) => {
console.log(clientX, clientY)
this.setState({
clientX,
clientY
})
})
}
render() {
const { children } = this.props
let childWithData = React.Children.map(children, c => {
if (!React.isValidElement(c)) {
return null
}
let cProps = {
...c.props,
clientX: this.state.clientX,
clientY: this.state.clientY
}
return React.cloneElement(c, cProps)
})
return childWithData ? childWithData : null
}
}
class Cat extends React.Component {
render() {
const { clientX, clientY } = this.props
return (
<img src="./cat.png" style={{ position: 'absolute', left: clientX - 64, top: clientY - 64 }} />
)
}
}
export default class App extends React.Component {
render() {
return (
<div>
<Mouse>
<Cat />
</Mouse>
</div>
)
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
结果
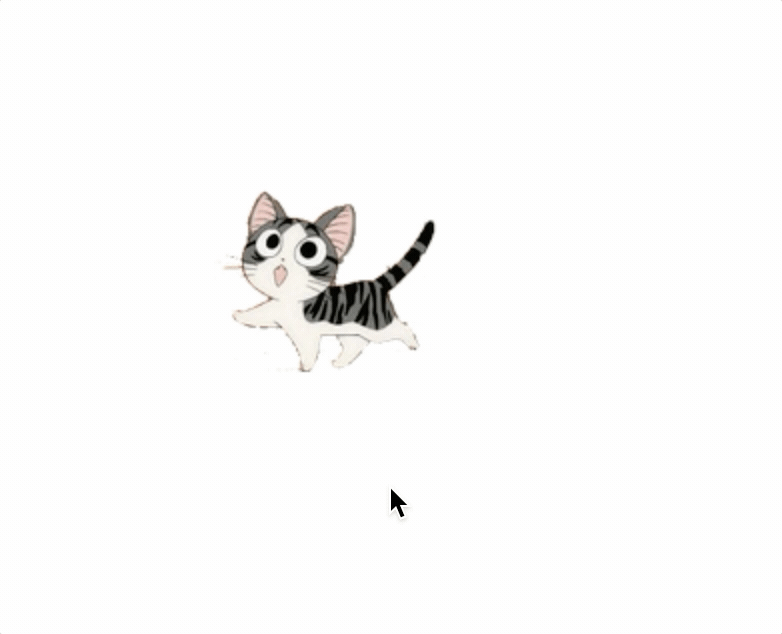