集合关联
Galloping_Leo 2020-01-21 MongoDB
# 集合关联
通常不同集合的数据之间是有关系的,例如文章信息和用户信息存储在不同集合中,但文章是某个用户发表的,要查询文章的所有信息包括发表用户,就需要用到集合关联。
# 基本操作
对于文章与作者数据之间的关系连接操作如下
- 使用
id
对集合进行关联 - 使用
populate
方法进行关联集合查询
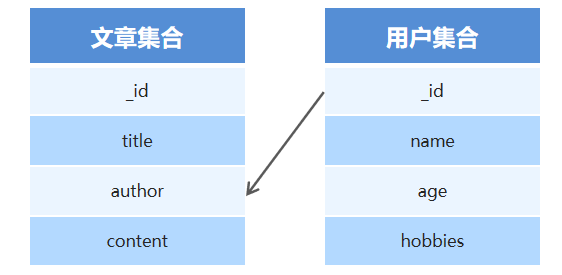
示例代码
//引入第三方数据模块
const mongoose = require('mongoose')
//创建数据库连接
mongoose.connect('mongodb://localhost/playground', { useNewUrlParser: true, useUnifiedTopology: true })
.then(result => console.log('连接成功!'))
.catch(err => console.log('连接失败!'))
const userSchema = new mongoose.Schema({
name: {
type: String,
required: true
}
})
const postSchema = new mongoose.Schema({
title: {
type: String
},
author: {
type: mongoose.Schema.Types.ObjectId,//类型设置为_id类型
ref: 'User' //关联User数据表
}
})
//用户集合构造函数
const User = mongoose.model('User', userSchema)
//文章集合构造函数
const Post = mongoose.model('Post', postSchema)
Post.find().populate('author').then(res => console.log(res))
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
# 关联
以上面代码为例,文章数据与用户数据是相关联的,文章中要指明是哪一个用户所写的,就必须把它与用户数据表相连接,在Post
的规则定义时,设置一个author
字段将其值设置为用户的id
值,这样两个数据集合就关联起来了。
author: {
type: mongoose.Schema.Types.ObjectId,//类型设置为_id类型
ref: 'User' //关联User数据表
}
1
2
3
4
2
3
4
# 查询
还是以上代码为例,使用Post.find()
方法会得到所有的结果,且结果的author
字段时id
值。
Post.find().then(res => console.log(res))
1
结果
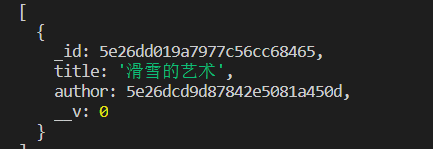
要想时查询结果的author
字段是对应id的用户详细信息,那么只需使用populate()
方法即可。populate()
方法中的参数为提供链接的字段(此处为author
)
Post.find().populate('author').then(res => console.log(res))
1
结果
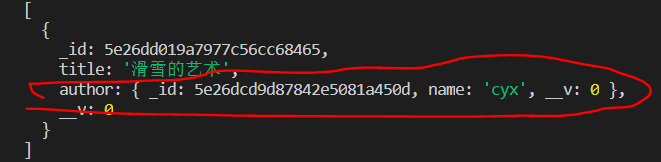